A Guide to Date & Time Management in Python
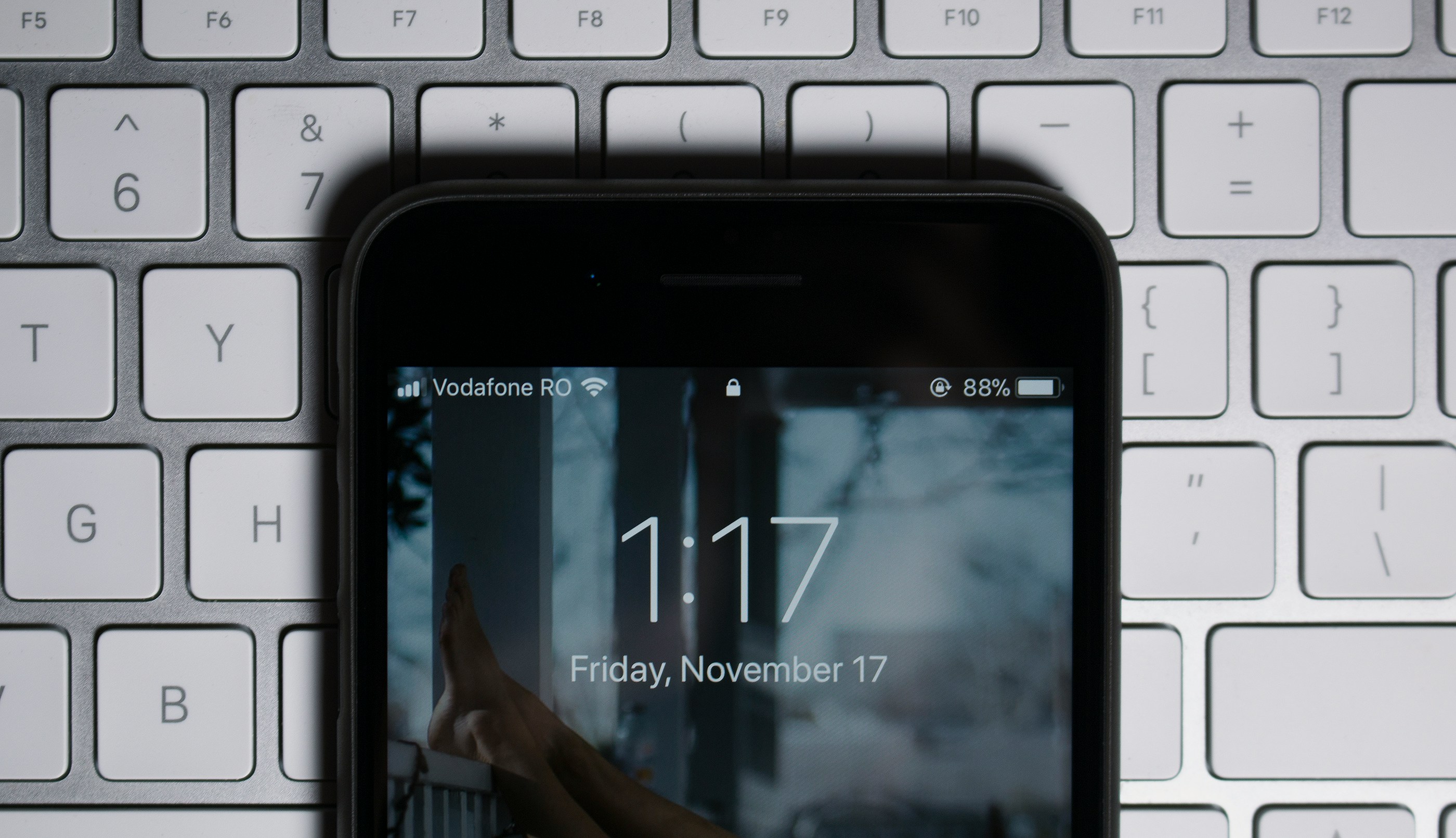
Introduction
Time—it’s something we take for granted in our daily lives, yet in the world of software development, handling it correctly can be one of the most challenging tasks. When building applications that serve users across different time zones, what seems like a simple concept quickly turns into a maze of edge cases, inconsistencies, and unexpected bugs.
Think about it: If a user schedules a meeting for 12:00 PM, what does that actually mean? Is it 12:00 PM in New York, London, or Tokyo? If an event is set to trigger at midnight, which midnight are we talking about? And if you’re working on a trading platform, how do you ensure that market opening and closing times are handled correctly, despite the fact that different exchanges operate in different time zones (and sometimes follow daylight saving time rules)?
Then there’s the real headache: date calculations. What happens when a user in New York (Eastern Time) creates an event at 11:30 PM on December 31st, but someone in Sydney (Australian Eastern Time) sees it as 1:30 PM on January 1st? That single event spans two different years! If your system isn’t designed properly, things like “daily reports” and “yearly summaries” can go terribly wrong.
And let’s not forget customer support issues—when a user reports a bug that happened at 2:00 PM their time, how do you figure out what that means in UTC, or on the server?
These are the types of challenges developers face when managing time and date logic in modern applications. Fortunately, Python provides a robust set of tools to help us navigate this minefield. In this guide, we’ll explore:
- The right way to store timestamps in a database to avoid confusion
- How to detect and manage the user’s time zone
- How to convert times accurately across different time zones
- The implications of daylight saving time (DST) and how to handle it
- How to schedule and trigger time-based events correctly
Problem Definition
Challenges with Time Zones
- Different time zones mean different actual times
- “Noon” in one timezone is not the same as “Noon” in another. This complicates scheduling and coordination.
- Date computations across time zones are tricky
- Adding or subtracting dates without considering time zones can yield incorrect results.
- Trading and other time-sensitive operations rely on market hours
- Markets operate on local time, which may include weekends and holidays that differ by region.
- Aggregation across time zones is challenging
- If a system needs to generate daily reports across time zones, determining what “today” means is not straightforward.
- Year crossover issues
- Users in different time zones may celebrate New Year at different times, impacting logic that depends on the year.
- Support complexity
- Users report issues based on their local time, while the system might log times in UTC. This requires proper conversion.
Focal Areas & Best Practices
To handle these challenges effectively, we need to focus on:
- Storage of dates
- Detecting and managing the client’s time zone
- Converting dates to a timezone-aware format
- Handling daylight saving time (DST)
- Triggering time-based events
1. Storage of Dates
Best Practice: Store all timestamps in UTC
To avoid inconsistencies, always store timestamps in Coordinated Universal Time (UTC) in your database. This ensures that conversions to local time zones remain accurate.
Python Implementation
Python’s datetime
module, along with pytz
or zoneinfo
, helps manage time zones correctly.
Example: Storing timestamps in UTC
1
2
3
4
5
6
from datetime import datetime, timezone
# Get the current time in UTC
utcNow = datetime.now(timezone.utc)
print(f"Current UTC time: {utcNow}")
Why store in UTC?
✅ Avoids ambiguity caused by daylight saving time (DST) changes
✅ Standardizes timestamps across different users
✅ Allows easy conversion to local time zones when needed
2. Detecting and Managing Client Time Zone
There are two ways to get the user’s time zone:
A. Detecting time zone automatically (Frontend Approach)
Most web applications rely on JavaScript to detect the user’s time zone and send it to the backend.
Example (JavaScript)
1
2
3
4
const userTimezone = Intl.DateTimeFormat().resolvedOptions().timeZone;
console.log("User Time Zone:", userTimezone);
// Send this timezone info to the backend
B. Allowing users to manually set their time zone
Some applications (e.g., scheduling apps) allow users to choose a preferred time zone. This setting should be stored in the database and used for conversions.
3. Converting Dates to a Timezone-Aware Representation
Once the user’s time zone is known, you need to convert stored UTC timestamps to the user’s local time.
Using pytz
(Third-party library)
1
2
3
4
5
6
7
8
9
10
11
from datetime import datetime
import pytz
# Assume this timestamp is fetched from the database (stored in UTC)
utcTime = datetime(2025, 3, 3, 12, 0, tzinfo=pytz.UTC)
# Convert to user's time zone (e.g., "Africa/Nairobi")
userTimezone = pytz.timezone("Africa/Nairobi")
localTime = utcTime.astimezone(userTimezone)
print(f"Local Time in Nairobi: {localTime}")
Using zoneinfo
(Built-in, recommended for Python 3.9+)
1
2
3
4
5
6
7
8
9
10
from datetime import datetime
from zoneinfo import ZoneInfo
# UTC time
utcTime = datetime(2025, 3, 3, 12, 0, tzinfo=ZoneInfo("UTC"))
# Convert to another timezone
localTime = utcTime.astimezone(ZoneInfo("Africa/Nairobi"))
print(f"Local Time in Nairobi: {localTime}")
Key Takeaways:
✅ Always use timezone-aware datetime
objects
✅ Use zoneinfo
(Python 3.9+) instead of pytz
where possible
4. Handling Daylight Saving Time (DST)
Problem: Some time zones shift due to DST
Certain regions (e.g., Morocco, Canary Islands, USA) observe DST, meaning clocks move forward or backward at specific times of the year.
Solution: Use timezone-aware datetime and libraries that handle DST automatically
Example: Handling DST with zoneinfo
1
2
3
4
5
6
7
8
9
10
from datetime import datetime
from zoneinfo import ZoneInfo
# A date before DST starts
dtBeforeDst = datetime(2025, 3, 10, 1, 30, tzinfo=ZoneInfo("Africa/Nairobi"))
print(f"Before DST: {dtBeforeDst}")
# A date after DST starts (clocks move forward by 1 hour on March 10, 2025)
dtAfterDst = datetime(2025, 3, 10, 3, 30, tzinfo=ZoneInfo("Africa/Nairobi"))
print(f"After DST: {dtAfterDst}")
✅ zoneinfo
ensures that the correct local time is displayed, even when DST changes.
5. Triggering Time-Based Events
Many applications require scheduling events based on time zones (e.g., a market closing at 5 PM local time).
Approach: Use UTC for event triggers and convert to local time as needed
Example: Checking if a market is open
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
from datetime import datetime
from zoneinfo import ZoneInfo
# Get current UTC time
utcNow = datetime.now(ZoneInfo("UTC"))
# Convert to Nairobi time
nrbTime = utcNow.astimezone(ZoneInfo("Africa/Nairobi"))
# Check if market is open (assuming 9:30 AM - 4:00 PM market hours)
marketOpen = datetime(nrbTime.year, nrbTime.month, nrbTime.day, 9, 30, tzinfo=ZoneInfo("Africa/Nairobi"))
marketClose = datetime(nrbTime.year, nrbTime.month, nrbTime.day, 16, 0, tzinfo=ZoneInfo("Africa/Nairobi"))
if marketOpen <= nrbTime <= marketClose:
print("Market is OPEN")
else:
print("Market is CLOSED")
Conclusion
Managing date and time across multiple time zones is a complex but essential aspect of modern applications. To avoid inconsistencies and ensure accuracy, it’s crucial to follow best practices:
✅ Always store timestamps in UTC – This provides a universal reference point, preventing confusion and errors when dealing with users in different time zones.
✅ Detect and store the user’s time zone – Whether automatically captured via the frontend or manually set by the user, storing this preference enables seamless conversions and accurate scheduling.
✅ Use timezone-aware datetime
objects – Libraries like zoneinfo
(Python 3.9+) and pytz
help ensure that your application correctly converts times while accounting for differences between regions.
✅ Consider daylight saving time (DST) shifts – Some regions adjust their clocks seasonally, which can impact scheduling and calculations. Using zoneinfo
ensures these changes are handled automatically.
✅ Trigger time-based events using UTC – Business logic, such as market opening and closing times, should be stored and processed in UTC, with appropriate conversions applied before presenting data to users.
By implementing these best practices, you can build a robust, timezone-aware system that provides accurate and reliable time-based functionality for all users, regardless of location. Whether you’re dealing with scheduling, trading hours, or global reports, careful handling of date and time ensures a smooth user experience.
Happy coding!