Optimizing Pattern Matching in F# for Better Performance
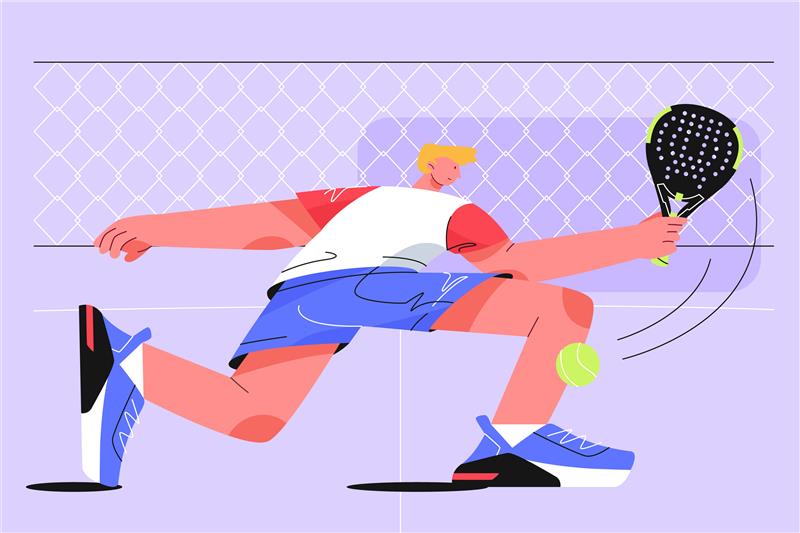
Pattern matching is one of the most powerful features of functional we can employ to ensure more expressive and easier-to-read code. However, handling large data structures may require optimal pattern matching to avoid performance. This article explores techniques to optimize pattern matching in F# by minimizing unnecessary computations and improving efficiency.
Common Performance Issues in Pattern Matching
Although pattern matching improves code readability, improper use can lead to inefficiencies. Some common issues include:
- Deep Nesting – Overly nested match expressions can make code hard to follow and lead to redundant evaluations.
- Unnecessary Conditions – Checking more cases than necessary increases execution time.
- Inefficient Data Structures – Poorly designed data representations can cause repetitive calculations.
- Repeated Pattern Checks – Evaluating the same values multiple times can create unnecessary overhead.
- Non-Exhaustive Matches – Missing cases may cause runtime errors and unexpected behavior.
- Non-Tail Recursive Functions – Functions without tail-call optimization can use excessive memory and degrade performance.
Let’s explore optimization strategies to address these issues and make pattern matching in F# more efficient.
1. Minimizing Nesting for Better Readability and Efficiency
Consider this recursive function that searches for the number zero in a list:
1
2
3
4
5
6
7
let rec processList lst =
match lst with
| [] -> "Empty list"
| x :: xs ->
match x with
| 0 -> "Zero found"
| _ -> processList xs
This function is deeply nested, making it harder to read and less efficient. We can rewrite it to be more concise:
1
2
3
4
let rec processList = function
| [] -> "Empty list"
| 0 :: _ -> "Zero found"
| _ :: xs -> processList xs
Flattening patterns in this way eliminates redundant computations and reduces unnecessary complexity.
2. Optimizing Conditions with Guard Expressions
Using unnecessary conditions can slow down execution. Take the following function:
1
2
3
4
5
let categorizeNumber n =
match n with
| 0 -> "Zero"
| x when x > 0 -> "Positive"
| x when x < 0 -> "Negative"
The condition x < 0
is redundant because it is the only remaining possibility. Instead, simplify it:
1
2
3
let categorizeNumber = function
| 0 -> "Zero"
| x -> if x > 0 then "Positive" else "Negative"
This reduces computation time by eliminating an unnecessary comparison.
3. Improving Recursion with Tail Call Optimization
Recursive functions can be memory-intensive if not optimized properly. Consider this non-tail-recursive sum function:
1
2
3
4
let rec sumList lst =
match lst with
| [] -> 0
| x :: xs -> x + sumList xs // Not tail-recursive
Each recursive call creates a new stack frame, which can lead to stack overflow for large lists. Instead, use tail recursion:
1
2
3
4
5
let sumList lst =
let rec loop acc = function
| [] -> acc
| x :: xs -> loop (acc + x) xs
loop 0 lst
This version accumulates the sum in acc
, making it more efficient and stack-friendly.
Leveraging fold
for Even Better Performance
For an even more optimized approach, F# provides List.fold
:
1
let sumList lst = List.fold (+) 0 lst
Using built-in functions like fold
is often the best approach since they are highly optimized for performance.
4. Using Active Patterns to Improve Readability and Performance
Active patterns allow complex pattern-matching logic to be encapsulated in reusable components. Consider categorizing strings based on their content:
1
2
3
4
5
6
7
8
9
10
let (|Number|Alpha|Other|) str =
if System.Int32.TryParse(str) |> fst then Number
elif str |> Seq.forall System.Char.IsLetter then Alpha
else Other
let categorize str =
match str with
| Number -> "Contains only numbers"
| Alpha -> "Contains only letters"
| Other -> "Mixed content"
This improves code modularity and readability while reducing repetitive logic. Active patterns can also be partial, like distinguishing even and odd numbers:
1
let (|Even|Odd|) x = if x % 2 = 0 then Even else Odd
Using active patterns simplifies code while maintaining efficiency.
5. Handling Large Data Structures Efficiently
For large-scale data structures, an optimized approach is crucial. Consider summing values in a binary tree:
1
2
3
4
5
6
7
type Tree =
| Leaf of int
| Node of Tree * Tree
let rec sumTree = function
| Leaf v -> v
| Node (left, right) -> sumTree left + sumTree right
While this recursive method is simple, deeply nested trees may cause performance issues. We could instead use an explicit stack-based traversal as below:
1
2
3
4
5
6
7
let sumTree tree =
let rec loop acc stack =
match stack with
| [] -> acc
| Leaf v :: rest -> loop (acc + v) rest
| Node (l, r) :: rest -> loop acc (l :: r :: rest)
loop 0 [tree]
This approach avoids deep recursion and prevents stack overflow while improving efficiency.
Efficient pattern matching is crucial for writing high-performance F# applications. The few examples above illustrate how we can optimize our functional code and build programs that are faster and more readable.
Additional performance improvements can come from techniques like memoization and parallel computation. Developers should continuously assess their pattern-matching logic to find the best balance between clarity and execution speed. This way they can create more efficient applications in areas such as financial modeling, data analysis, and large-scale algorithmic computing, ensuring better performance while maintaining functional programming principles.